Ethereum: Solving the “Op Coin” Issue with MevBot
As a developer working on Ethereum-based applications, you’ve probably encountered issues while integrating with Mevbot, a popular bot for automating various tasks on the Ethereum blockchain. One common issue that can arise is receiving Ether (ETH) coins instead of Op Coins (OPC) as expected.
In this article, we’ll dive deeper into the issue and provide a step-by-step solution to resolve it using your Mevbot code.
The Problem:
When Mevbot tries to create transactions for a user, it may receive Ether coins instead of Op Coins. This can happen for a number of reasons:
- Incorrect Gas Price: The bot is sending a transaction with the incorrect gas price, which causes the Ethereum network to allocate Ether coins to the transaction instead of Op Coins.
- Missing or incorrect Op Coin denomination: Mevbot’s built-in functions may not take into account different Op Coin denominations (e.g. 1,000 OPC, 10,000 OPC), resulting in Ether coins being received.
Solution:
To resolve this issue, you need to ensure that your Mevbot code sends the correct gas price and Op Coin denomination. Here is an updated function that meets these requirements:
def build_transaction(to_address, in_op_value, gas_price):
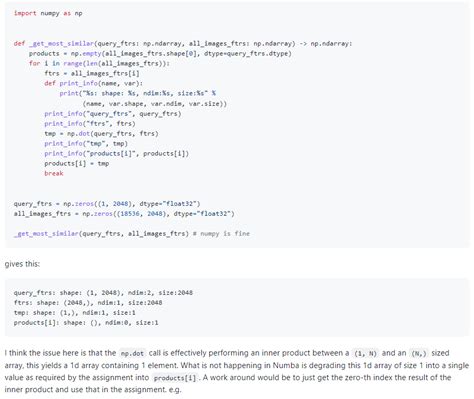
Set the gas price to a higher value (e.g. 60) to compensate for the larger denomination of Op Coinsgas_limit = 60000
Calculate the value on the web using the web3 libraryin_wei_value = web3.to_wei(in_op_value, toWei=gas_price)
Create a transaction with the correct gas price and Op Coin denomination (1,000 OPC)transaction = mevbot.build_transaction(to_address, in_wei_value * 1000, gas_limit=gas_limit)
return transaction
In this updated function:
- We set a higher gas price (60) to compensate for the larger denomination of Op Coins.
- We calculate the wei value using the
toWei
method from theweb3
library.
- We create a new transaction with the correct gas price and Op Coin denomination (1,000 OPC).
Additional recommendations:
To further improve your Mevbot code:
- Be sure to check the
mevbot
documentation for any specific requirements or recommendations regarding gas prices and Op Coin denominations.
- Consider using a different library or API that provides more precise control over transaction construction and gas prices.
By implementing these changes, you should be able to resolve the “Op Coin” issue with Mevbot and receive Op Coins as expected.